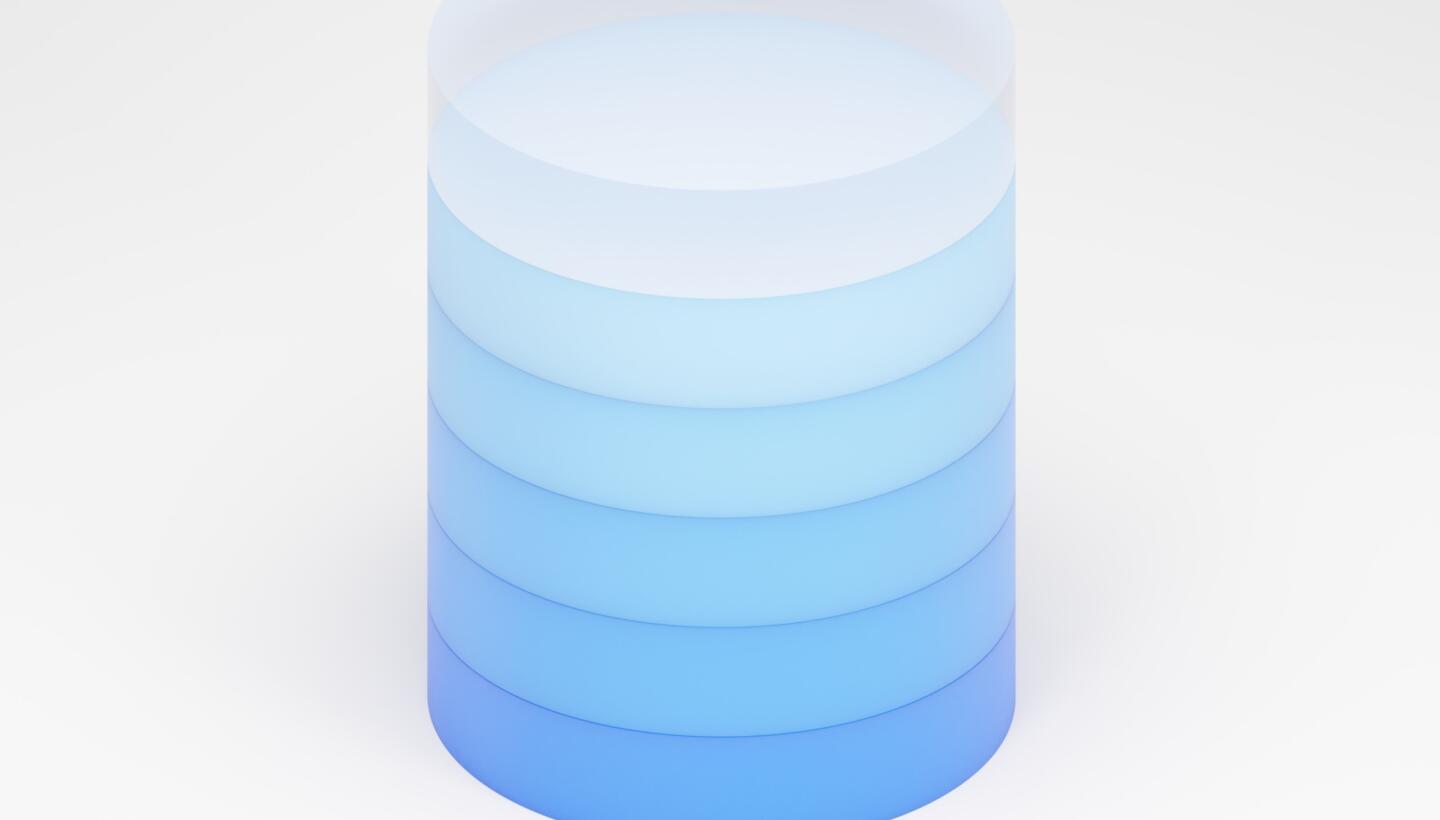
8 Techniques for Optimizing SQL Queries
In this article, we will outline techniques for optimizing SQL queries to improve performance.
Indexes
Indexes speed up data retrieval by creating a separate data structure that allows for faster searching. They should be created on frequently queried columns to enable the database to quickly locate the data needed to fulfil a query. Having too many indexes can negatively impact performance by increasing the time it takes to insert and update data. Therefore, it's important to create indexes only on frequently searched columns.
CREATE INDEX index_name ON table_name(column_name);
Joins
Joining tables in SQL is a technique for combining data from multiple tables into a single result set. Poorly written join queries can lead to slow performance. One way to optimize join queries is to use INNER JOIN instead of OUTER JOIN, as INNER JOIN only returns rows that have matching values in both tables. Additionally, using subqueries instead of joins can sometimes result in better performance, as subqueries retrieve only the necessary data.
SELECT * FROM table1 INNER JOIN table2 ON table1.id = table2.table1_id WHERE table1.id = 1;
Selecting Columns
When querying a database, it is important to select only the columns necessary for the task at hand. Selecting unnecessary columns can lead to slower performance and increased memory usage. Therefore, it's important to select only the columns needed for a specific query.
By using these techniques, you can improve the speed and efficiency of your SQL queries. Additionally, there are several other techniques you can use to optimize SQL queries, such as using WHERE and HAVING clauses, limiting results, using stored procedures, and more.
SELECT column1, column2 FROM table_name;;
Using WHERE and HAVING Clauses
The WHERE and HAVING clauses filter data based on specific conditions. By using these clauses, the database can retrieve only the necessary data, resulting in faster performance. Additionally, using these clauses in combination with indexes can further improve query performance.
SELECT * FROM table_name WHERE column_name = 'value' HAVING COUNT(column_name) > 1;
Limiting Results
Limiting the number of results returned by a query can significantly improve performance, especially when working with large databases. Using the LIMIT clause can limit the number of rows returned by a query, while the TOP clause can be used in SQL Server to limit the number of rows returned.
SELECT * FROM table_name LIMIT 10;
Using Stored Procedures
Stored procedures are precompiled SQL statements that can be executed multiple times with different parameters. By using stored procedures, you can reduce the overhead associated with compiling and optimizing the SQL query each time it is executed.
CREATE PROCEDURE procedure_name(IN parameter1 INT, IN parameter2 VARCHAR(50)) BEGIN -- SQL statements here END; -- Example call to stored procedure CALL procedure_name(1, 'value');
Use EXISTS() instead of COUNT() to Find a Specific Element in the Table
When trying to determine whether a specific element is present in a table, it can be tempting to use the COUNT() function to count the number of occurrences of that element. However, using EXISTS() can be a more efficient approach, as it allows the database to stop searching for the element as soon as it is found. This can be particularly useful when working with large databases.
SELECT * FROM table_name WHERE EXISTS(SELECT * FROM table_name WHERE column_name = 'value');
Use VARCHAR Instead of CHAR for Character Strings
Both VARCHAR and CHAR data types are used to store character strings in SQL. However, VARCHAR is generally more efficient than CHAR, as it only uses the necessary amount of storage space for each string, while CHAR reserves a fixed amount of space for each string, even if the string is shorter. Therefore, it is generally best to use VARCHAR for storing character strings, unless there is a specific reason to use CHAR.
CREATE TABLE table_name id INT PRIMARY KEY, charCol CHAR(10), varcharCol VARCHAR(10) );
Publicerat av: